A custom made search field with selectable search suggestions.
Dart
x
93
93
1
import 'package:flutter/material.dart';
2
3
class Searching extends StatefulWidget {
4
5
_SearchingState createState() => _SearchingState();
6
}
7
8
class _SearchingState extends State<Searching> {
9
TextEditingController editingController = TextEditingController();
10
11
final _items = List<String>.generate(100, (i) => "Søgetekst $i");
12
List<String> _itemsSuggestions = [];
13
14
void searchResults(String query) {
15
setState(() {
16
_itemsSuggestions = _items
17
.where((data) => data.toLowerCase().contains(query.toLowerCase()))
18
.toList();
19
});
20
}
21
22
23
Widget build(BuildContext context) {
24
return Scaffold(
25
appBar: AppBar(
26
title: Text('Søgning'),
27
),
28
body: Container(
29
child: Column(
30
children: <Widget>[
31
Padding(
32
padding: const EdgeInsets.all(8.0),
33
child: TextField(
34
onChanged: (value) => searchResults(value),
35
onSubmitted: (value) {
36
setState(() {
37
_itemsSuggestions = [];
38
});
39
print(value);
40
},
41
controller: editingController,
42
decoration: InputDecoration(
43
isDense: true,
44
labelText: "Søg efter varer",
45
hintText: "Varenavn",
46
prefixIcon: IconButton(
47
icon: Icon(Icons.arrow_back),
48
onPressed: () {},
49
),
50
suffixIcon: IconButton(
51
icon: Icon(Icons.clear),
52
onPressed: () {
53
setState(() {
54
editingController.text = '';
55
});
56
}),
57
border: OutlineInputBorder(
58
borderRadius: BorderRadius.all(
59
Radius.circular(10.0),
60
),
61
),
62
),
63
),
64
),
65
Expanded(
66
child: ListView.builder(
67
itemCount: _itemsSuggestions.length,
68
itemBuilder: (context, index) {
69
return ListTile(
70
dense: true,
71
leading: Icon(Icons.search, color: Colors.grey,),
72
title: Text(
73
'${_itemsSuggestions[index]}',
74
style: TextStyle(
75
color: Colors.grey,
76
fontSize: 16,
77
),),
78
onTap: () {
79
setState(() {
80
editingController.text = _itemsSuggestions[index];
81
});
82
},
83
);
84
},
85
),
86
),
87
],
88
),
89
),
90
);
91
}
92
}
93
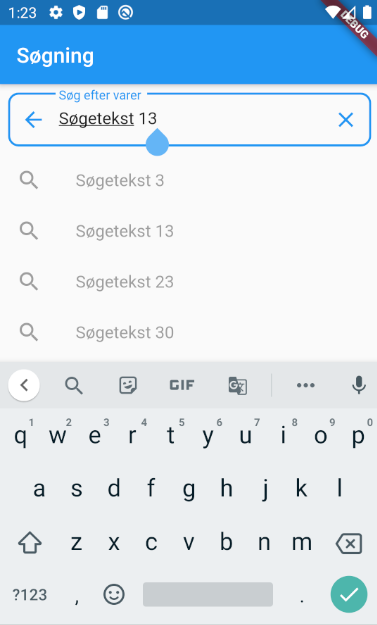